Ensuring that a product meets the stakeholders’ requirements or expectations is crucial in the software development process. To achieve that, we need someone in our team to regularly verify and validate the product.
Do you know whose role this belongs to?
You guessed it; the quality assurance (QA) team!
When it comes to running tests, it is definitely possible for the QA team to do manual testing when a product is still relatively small. However, as a product grows, QA will eventually only have time to test some of the features for each new release.
In this article, we will discuss about using test automation to tackle this challenge and how to conduct it.
What is Test Automation?
Test automation is automating the test execution and determining the pass or fail of the test through some software or script other than the software being tested. The primary purpose of automation is to save time and effort on repetitive tasks.
There are a lot of tools that you can use to perform test automation.
Open-Source Tools | Commercially–Licensed Tools |
Selenium | WatiN |
Appium | Katalon Studio |
TestNG | Eggplant |
Robotium | TestComplete |
Cucumber | Testsigma |
Watir | Testlink |
Sikuli |
Selenium
The most commonly used test automation tool is Selenium, which is widely used by many QA teams in the industry. Selenium is an umbrella project for a range of tools and libraries that enable and support the test automation on web browsers. Selenium is exclusively for web-based applications and supports multiple browsers, platforms, and languages.
Selenium includes a product named Selenium WebDriver, which helps to create robust browser-based regression automation suites and tests by using automation APIs to control browsers and run tests.
Benefits of Using Selenium
There are many advantages to using Selenium as your automated test tool.
1. Language and Framework Support
Selenium supports all major languages like Java, Python, JavaScript, C#, Ruby, and Perl programming.
2. Open-Source Availability
Being an open-source tool, Selenium is a publicly accessible automation framework that’s free of charge! So, you can save your money and use them for other good causes.
3. Multi-Browser Support
Chrome, Firefox, Safari, Internet Explorer, Opera, and Edge browsers are some of the most used browsers worldwide. The Selenium script is compatible with all of the aforementioned browsers. You don’t need to rewrite scripts for every browser, just one script for all browsers.
4. Support Across Various Operating Systems
Selenium is a highly portable tool that supports and can work across different operating systems like Windows, Linux, Mac OS, UNIX, etc.
5. Ease of Implementation
Selenium automation framework is a very easy-to-use tool. Selenium provides a user-friendly interface that helps create and execute test scripts effectively. You can also watch while tests are running, analyze detailed reports of Selenium tests, and take follow-up actions.
6. Reusability and Integrations
Selenium test automation suites are reusable and can be tested across multiple browsers and operating systems. However, it needs third-party frameworks and add-ons to broaden the scope of testing. For example, you must integrate Selenium with TestNG and JUnit to manage test cases and generate reports. To achieve continuous testing, you must integrate it with CI/CD tools like Jenkins, Maven, and Docker.
7. Flexibility
Test management becomes easier and more efficient with Selenium features like regrouping and refactoring test cases. This helps developers and testers make quick code changes, reducing duplication, minimizing complications, and improving maintainability. These features make Selenium more flexible and usable compared to other test automation tools, which helps Selenium to keep an edge.
8. Parallel Test Execution and Faster Go-to-Market
With the help of Selenium Grid, we can execute multiple tests in parallel, reducing the test execution time. With the help of cloud grids for cross-browser testing, you can test across as many browsers in parallel using Selenium, saving you plenty of time.
9. Less Hardware Usage
If you compare Selenium with other vendor-focused automation tools like QTP, UFT, and SilkTest, you will find that Selenium requires less hardware resources than other testing tools.
10. Easy to Learn and Use
Writing Selenium scripts is not more than writing a few pieces of code to automate your website’s functionalities. Also, documentation on the Selenium website is beneficial for developers and testers to start with Selenium test automation.
11. Constant Updates
Since Selenium is supported by a community (and we all know that an active community doesn’t like to stay stagnant), the Selenium community is constantly releasing updates and upgrades. The best part about having a community is that these upgrades are readily available and easy to understand. Therefore, you do not need any specific training. This makes Selenium resourceful compared to other tools and not to mention cost-effective!
Starting Test Automation
Firstly, we need to set up the configuration for test automation.
The steps are:
- Install Java and set Java Home Path in system variables.
- Install Eclipse and create a new Maven project with Selenium dependencies.
- Understand the basics of the WebDriver commands.
- Run the Selenium WebDriver automation program.
Next, navigate to this page and download any JDK version from 8 upwards to run Selenium Test by following the installation instructions.
Once you have finished the installation process, you must set Java Home Path in system variables.
Refer to this instruction to navigate to the environment variables page:
Control Panel > System and Security > System > Advanced System Setting > Environment Variables.
Then add variable name: JAVA_HOME and variable value: [location of your JDK directory]
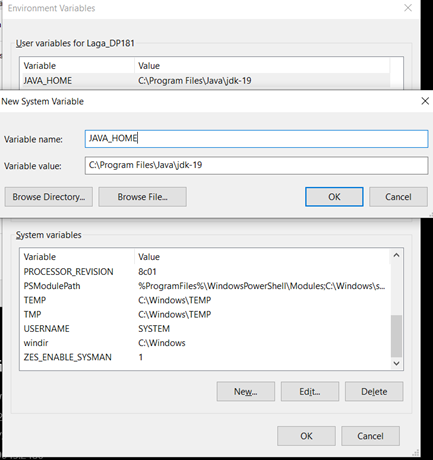
Next, you must install Eclipse on your device. To install Eclipse go to this page and follow the installation instructions. After you have finished the installation, create a new Maven project.
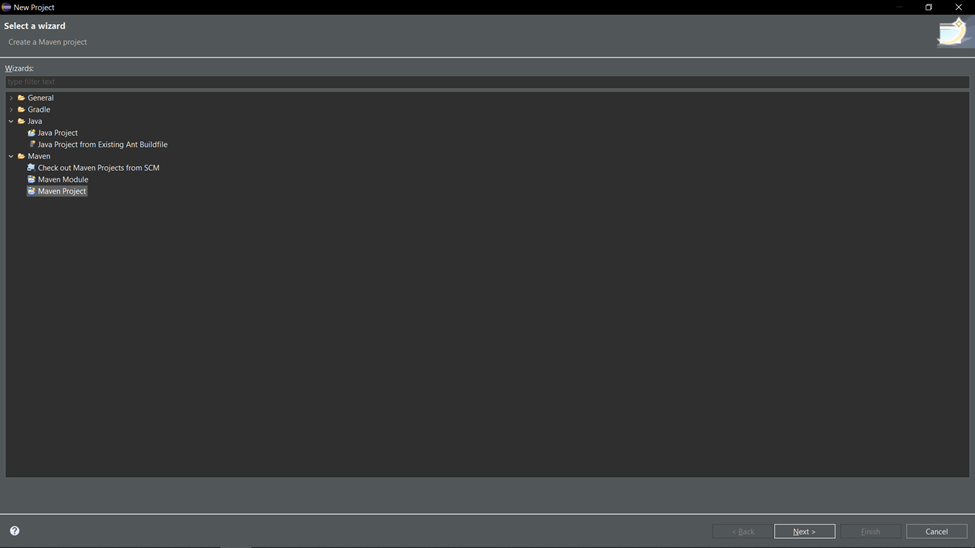
In pom.xml, add the Selenium and TestNG dependencies, which you can find in the links below:
https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium
java/4.7.2https://mvnrepository.com/artifact/org.testng/testng/7.7.0
Make sure to save the updated Maven project. Doing so applies the dependencies to your project, so do not skip this step!
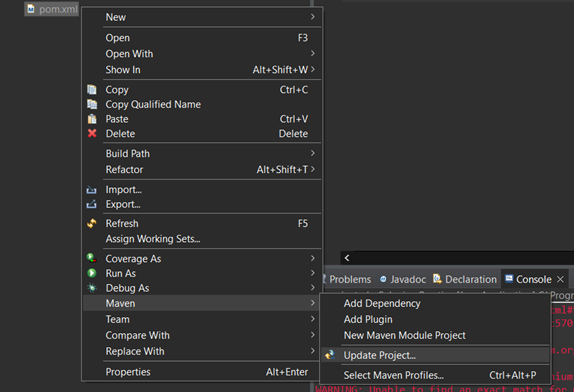
The code will then look like this:
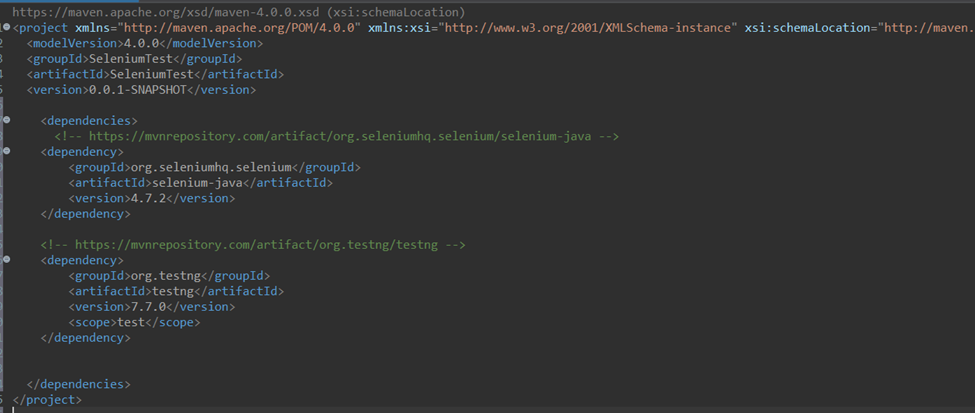
Next, we need to run the configuration by using TestNG. Go to the help menu > eclipse marketplace > search TestNG.
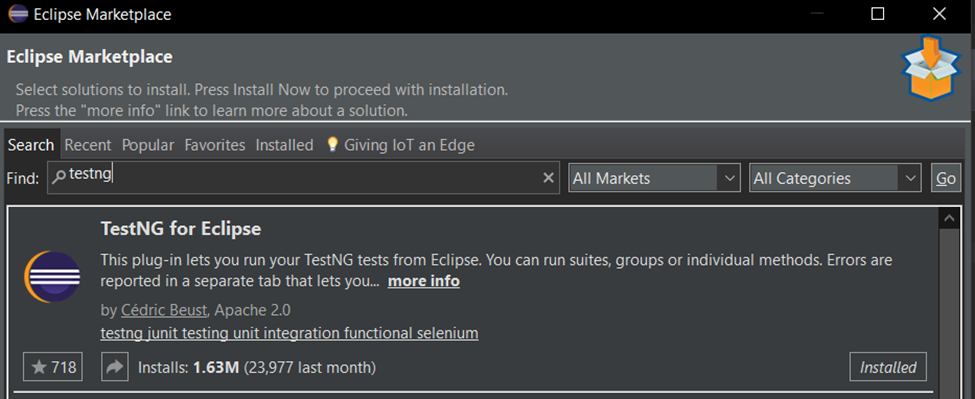
Then create a Java class named “SeleniumPractice” and click finish.
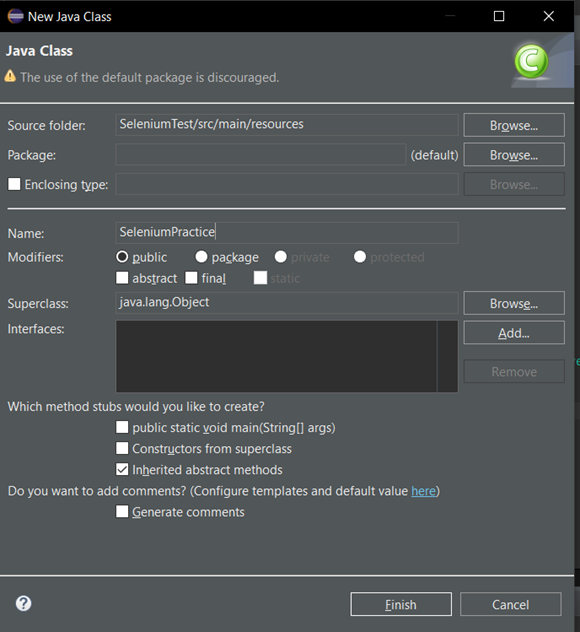
Download the Chrome WebDriver from this page and put it under src/main/resources.
Now, change the system properties directly into the ChromeDriver file, then add the web driver declaration. As we are using the Chrome WebDriver, we declare the “driver” variables with “ChromeDriver()”
If you are using another browser web driver, you can configure it accordingly.
There are 3 components to basic test automation:
- Locator: to locate a web element, use find by XPath, id, class name, etc. You will need to use the one that works on the web pages.
- Action: Selenium provides actions like sending keys, clicking, getting URLs, titles, etc. It would help if you used the action component to create your test scripts.
- Assertion: this component compares the actual result and the expected result.
Here are some simple examples of combining the locator, action, and assertion. Before running the code, ensure all required setup is already done to avoid any errors.
1.
2. import java.sql.Driver;
3. import java.time.Duration;
4. import org.openqa.selenium.By;
5. import org.openqa.selenium.WebDriver;
6. import org.openqa.selenium.WebElement;
7. import org.openqa.selenium.chrome.ChromeDriver;
8. import org.testng.Assert;
9. import org.testng.annotations.AfterTest;
10. import org.testng.annotations.BeforeTest;
11. import org.testng.annotations.Test;
12.
13. public class SeleniumPractice{
14. WebDriver driver;
15. String userName= "standard_user";
16. String password = "secret_sauce";
17. @BeforeTest
18. public void InitDriver() {
19. System.setProperty("webdriver.chrome.driver", "src\\main\\resources\\chromedriver.exe");
20. driver = new ChromeDriver();
21. driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
22. driver.get("https://www.saucedemo.com/");
23. driver.manage().window().maximize();
24. }
25.
26. @Test(priority=1)
27. public void CheckUserNameLoginElement() {
28. WebElement userNameField = driver.findElement(By.xpath("//*[@id=\"user-name\"]"));
29. Assert.assertNotNull(userNameField);
30. }
31.
32. @Test(priority=2)
33. public void CheckPasswordLoginElement() {
34. WebElement passwordField = driver.findElement(By.name("password"));
35. Assert.assertNotNull(passwordField);
36. }
37.
38. @Test(priority=3)
39. public void CheckButtonLoginElement() {
40. WebElement loginButton = driver.findElement(By.className("btn_action"));
41. Assert.assertNotNull(loginButton);
42. }
43.
44. @Test(priority=4)
45. public void CheckLoginValidUser() {
46. WebElement userNameField = driver.findElement(By.id("user-name"));
47. WebElement passwordField = driver.findElement(By.id("password"));
48. WebElement loginButton = driver.findElement(By.id("login-button"));
49. driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
50. userNameField.sendKeys(userName);
51. passwordField.sendKeys(password);
52. loginButton.click();
53. String url = driver.getCurrentUrl().toString();
54. Assert.assertEquals(url, "https://www.saucedemo.com/inventory.html");
55. }
56.
57.
58. @AfterTest
59. public void close() {
60. driver.close();
61. driver.quit();
62. }
63.
64. }
When you run the script, and the browser test pops up automatically, it will run the command based on priority. First, the browser will execute “CheckUserNameLoginElement” then “CheckPasswordLoginElement” and will continue until “CheckLoginValidUser”
The result of this scenario will show on the console log. There are 3 types of results: pass, failure, and skip.
As you can see, we have 4 scenarios, all of which have passed. So, this is a good indicator.
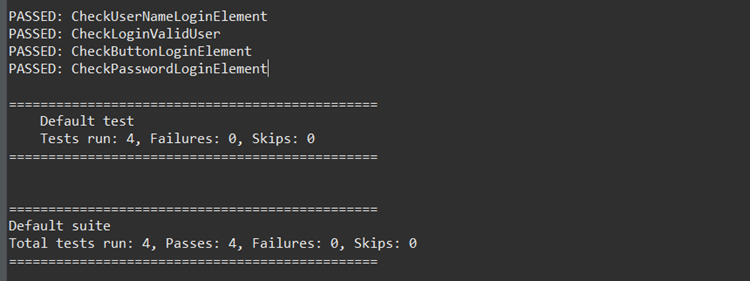
Now let’s try adding a negative case.
8. import java.sql.Driver;
9. import java.time.Duration;
10. import org.openqa.selenium.By;
11. import org.openqa.selenium.WebDriver;
12. import org.openqa.selenium.WebElement;
13. import org.openqa.selenium.chrome.ChromeDriver;
14. import org.testng.Assert;
15. import org.testng.annotations.AfterTest;
16. import org.testng.annotations.BeforeTest;
17. import org.testng.annotations.Test;
18.
19. public class SeleniumPractice{
20. WebDriver driver;
21. String userName= "standard_user";
22. String password= "secret_sauce";
23. String wrongUserName= "testWrongUser";
24. String WrongPassword= "testWrongPass";
25. @BeforeTest
26. public void InitDriver() {
27. System.setProperty("webdriver.chrome.driver", "src\\main\\resources\\chromedriver.exe");
28. driver = new ChromeDriver();
29. driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
30. driver.get("https://www.saucedemo.com/");
31. driver.manage().window().maximize();
32. }
33.
34. @Test(priority=1)
35. public void CheckUserNameLoginElement() {
36. WebElement userNameField = driver.findElement(By.xpath("//*[@id=\"user-name\"]"));
37. Assert.assertNotNull(userNameField);
38. }
39.
40. @Test(priority=2)
41. public void CheckPasswordLoginElement() {
42. WebElement passwordField = driver.findElement(By.name("password"));
43. Assert.assertNotNull(passwordField);
44. }
45.
46. @Test(priority=3)
47. public void CheckButtonLoginElement() {
48. WebElement loginButton = driver.findElement(By.className("btn_action"));
49. Assert.assertNotNull(loginButton);
50. }
51.
52. @Test(priority=4)
53. public void CheckLoginInvalidUser() throws InterruptedException {
54. WebElement userNameField = driver.findElement(By.id("user-name"));
55. WebElement passwordField = driver.findElement(By.id("password"));
56. WebElement loginButton = driver.findElement(By.id("login-button"));
57.
58. userNameField.sendKeys(wrongUserName);
59. passwordField.sendKeys(WrongPassword);
60. loginButton.click();
61. Thread.sleep(1000);
62. WebElement errorMesagElement = driver.findElement(By.xpath("/html/body/div[1]/div/div[2]/div[1]/div[1]/div/form/div[3]/h3"));
63.
64.
65. Assert.assertEquals(errorMesagElement.getText(), "Epic sadface: Username and password do not match any user in this service");
66. }
67.
68. @Test(priority=5)
69. public void CheckLoginValidUser() throws InterruptedException {
70. WebElement userNameField = driver.findElement(By.id("user-name"));
71. WebElement passwordField = driver.findElement(By.id("password"));
72. WebElement loginButton = driver.findElement(By.id("login-button"));
73. driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
74. userNameField.clear();
75. userNameField.sendKeys(userName);
76. Thread.sleep(1000);
77. passwordField.clear();
78. passwordField.sendKeys(password);
79. loginButton.click();
80. Thread.sleep(1000);
81. String url = driver.getCurrentUrl().toString();
82. Assert.assertEquals(url, "https://www.saucedemo.com/inventory.html");
83.
84. }
85.
86. @AfterTest
87. public void close() {
88. driver.close();
89. driver.quit();
90. }
91.
92. }
The results will look like this:
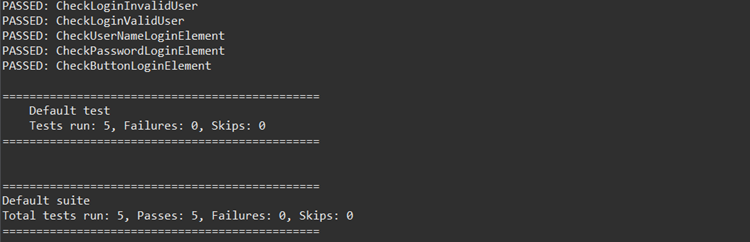
And that’s it!
Next, you can try another test case by combining 3 of the components to make more complex test suites.
Author: I Kadek Laga Dwi Pandika, Software Quality Tester